Archive for category Uncategorized
Duetto (C++ for the Web) 0.9.4: Async RPCs, Promises, JavaScript interoperability
Posted by Alessandro Pignotti in Uncategorized on March 17, 2014
Around a month has passed since our talk at mloc.js. There we had a great opportunity to talk about the technology behind the duetto C++ compiler for the Web. We also made our first public announcement of our promise based support for asynchronous type safe RPC, which fits in our vision of C++ as the platform language for the Web, both on the client and on the server side.
Today, we announce the new, feature-packed release of Duetto — 0.9.4! This time, we really splurged, and added some quality features that we’re sure you’ll find interesting:
-
Initial support for promise-based async RPC
-
Strong interoperability with JavaScript
-
__asm__ to execute JavaScript from C++ code
-
[[jsexport]] attribute to use C++ objects from JavaScript
-
-
Support for C++ mutex/atomic/thread headers
As usual, you can find the full source code of the release here, plus binary packages for Debian/Ubuntu on our PPA, a binary setup for windows and a binary archive for Mac OS X here.
Asynchronous RPC using promises
With duetto you can “tag” a method to let the compiler know that the code should be compiled for the server side. The compiler also takes care of generating RPC boilerplate including type safe serialization of parameters and deserialization of the return value. While the basic model is synchronous, you can take advantage of promises to use fully type safe asynchronous RPC in duetto. See e.g. the following code:
// Pending requests for messages
vector<Promise<string>*> pendingRequests;
Promise<string>* getChatMessageRemote() [[server]]
{
auto ret=new Promise<string>();
// Store the new promise, you can use it later to complete
// the request in asynchronous manner
pendingRequests.push_back(ret);
return ret;
}
void sendMessageRemote(const string& str) [[server]]
{
for (auto p: pendingRequests)
p->done(str);
pendingRequests.clear();
}
void messageHandler(const string& newMessage) [[client]]
{
client::console.log("Message received",newMessage.c_str());
}
void webMain() [[client]]
{
// First wait for messages
auto promise = getChatMessageRemote();
// Add a callback to the promise
promise->then(messageHandler);
// Tell the promise that no more callbacks will be added
promise->complete();
// Now send a message, it will be echoed back
sendMessageRemote("Test message");
}
The code is a simple but working example of a multi-user chat application. The getChatMessageRemote method returns a promise immediately after being called, without waiting for the server answer. On the server side we create a promise and return it. We also need to store it somewhere to eventually fulfill the promise by sending a value.
The client code needs to set a callback to receive the value when the promise will be eventually fulfilled. Later on the client send a chat message using the sendMessageRemote server method. This method is synchronous and will return only after the server side execution is completed. The server echoes the message back to all connected clients by fulfilling all pending promises.
Strong JS interoperability
This release of duetto includes two features that provide a superior integration with manually written JavaScript code: the [[jsexport]] class attribute and __asm__ support for inline JavaScript.
[[jsexport]] class attribute
We have introduced preliminary support for a new attribute: [[jsexport]]. This attribute is designed to be applied on C++ classes, doing so will cause make the class available to manually written JavaScript code. The following example shows its typical use:
class [[jsexport]] ExportExample
{
private:
float a;
int b;
public:
ExportExample(float _a, int _b):a(_a),b(_b)
{
}
void testMethod()
{
client::console.log(“Float value is”, a);
}
};
During compilation duetto will generate bridge code to expose the ExportExample C++ class to JavaScript code. You can then use the class when writing JS code
// Using jsexport-ed classes from JavaScript code
var cppobj = new ExportExample(0.1, 42);
cppobj.testMethod();
The [[jexport]] attribute places some limitations on the class. In particular, the object must have a trivial destructor and no overloaded methods. Moreover operators can’t be exported to JavaScript. Having a trivial destructor is necessary to make sure that the regular Garbage Collection mechanism is sufficient to reclaim the object. We plan to expand this feature in the future to reduce any limitations as much as possible.
Inline JavaScript using __asm__
This release also adds support for inserting arbitrary JavaScript code inside C++ code using the __asm__ functionality. Our design choice has been to put no limit on what you can do inside __asm__, including breaking the program, so be careful when taking advantage of this capability.
void webMain()
{
__asm__(“alert(‘JavaScript code inside __asm__’);”);
}
At the moment only this simple form of JS code insertion is supported. It’s not currently possible to pass variables from surrounding C++ code or return a value from __asm__, but we will extend this support in the future.
Support for C++ mutex/atomic/thread headers
Some C++ functionalities, especially the ones related to multi-threading, do not have a direct mapping on the browser platform. That said, we want to provide as much compatibility as possible with existing C++ code to reduce the effort when using duetto for porting.
As of duetto 0.9.4, we support the following C++ threading primitives:
-
mutex: Implemented as plain counters, mutexes cannot block execution but report an error if a non-recursive mutex is acquired twice.
-
atomic: Implemented as plain integers, since the browser is not actually concurrent there is no real support for atomic operations.
-
thread: Modern browsers support WebWorkers, but the threading model does not map to the one expected by C++ threads. We chose to make the user aware that C++ threads are not supported by adding an explicit error message when the thread header is included in code compiled with duetto.
Follow us on @leaningtech, Facebook and at www.leaningtech.com for updates.
Duetto: a C++ compiler for the Web going beyond emscripten and node.js
Posted by Alessandro Pignotti in Uncategorized on October 31, 2013
Today Leaning Technologies Ltd. releases duetto, a compiler designed to develop integrated (backend and frontend) web applications in C++. duetto is now available to the public as FOSS here, and will be offered in a commercial package with closed-source-friendly licensing soon.
Browser-based applications will undoubtedly play a big role in the future of both consumer and business applications. Web apps have some clear advantages compared to native applications, like being natively cloud-based and collaborative, while also being inherently portable, especially thanks to the increased attention that browser vendors have dedicated to web standard compliancy. Modern web standards, that usually go under the umbrella term HTML5, provide incredible close-to-native capabilities and this gap will be shrinking with time.
Several tools have been developed to help programmers in writing large-scale Web application frontends, reducing the limitations and obstacles given by Javascript: CoffeScript, Microsoft TypeScript, Google Dart, Google GWT and, more recently, emscripten, which compiles LLVM bytecode (and therefore C++) to Javascript. On the server side, it is common to use languages such as PHP, Python and Ruby, leading to a separate backend codebase in a language other than Javascript. This causes duplication of code (and new bugs), having to deal with RPC manually, and overall takes time away from proper development. A very popular solution for this has been to use Javascript on the server as well with node.js.
We present duetto, our C++ compiler for the Web, which is now available to the public. Our solution integrates and surpasses features of existing tools, and allows to program both the frontend and the backend of a Web application in an integrated C++ codebase, compiling them respectively to JavaScript and native code.
Duetto combines the advantages of emscripten and node.js by allowing the programmer to:
-
write web applications in C++, reusing existing code and making porting of whole applications and games to the browser plausible.
-
code both the frontend and the backend of a web application in the same language and codebase
In addition to this, duetto provides some nice features:
-
Bring the robustness and proven scalability of C++ programming to the Web
-
You can access all browser APIs directly. Duetto inherits the C++ philosophy of exposing the platform capabilities (and limitations) to the users. There is no middle man.
-
Duetto is based on LLVM/clang. An industry standard C++ compiler is a programmer’s best friend: code sanity is verified as compile time, including RPC signatures.
-
The LLVM toolchain also guarantees that a mind-blowing set of optimizations is run at compile time, generating highly efficient code. This decreases the burden on JavaScript JIT compiler at runtime.
-
Contrarily to emscripten we do not try to emulate a traditional address space using typed arrays, but directly map C++ objects to JS objects. This reduces memory consumption since the garbage collector can delete unused object.
Are you interested in using duetto? You can now. We have just released the whole technology as a FOSS project, available here. Also, we’ve stuck to our promise of releasing in six months from our first announcement in April
The compiler itself, derived from LLVM/clang is released with the same license as LLVM/clang (UI/NCSA) and we plan to contribute generally useful code upstream. Accompanying headers and libraries will be released as GPLv2+. We will be also offering closed source friendly licensing of such components for a fee. Here are links to the various repositories on github:
-
duetto enabled LLVM
-
duetto enabled clang
-
duetto headers and utilities
Release tarballs will be available shortly on the Launchpad page of the project: https://launchpad.net/duetto. Launchpad will also be our bug reporting system, so please use it to report any problem you might find. We will be also providing builds of duetto for Ubuntu linux using a PPA. We plan to provide binary builds for Mac OS X and Windows as well, but we are still working on automating those build.
Please subscribe to our Launchpad group/mailing list to discuss bugs, desired features and usability issues on https://launchpad.net/~duetto-users. You can also follow us on twitter (@leaningtech) and visit our website to be updated on duetto related news:
Linux support for Asus Xonar U1 USB audio device
Posted by Alessandro Pignotti in Uncategorized on January 15, 2013
I got as a Christmas gift an external USB audio device: an Asus Xonar U1. It’s a nice device with a decent audio quality. The audio itself, both in and out works perfectly with the standard snd-usb-audio kernel module. Unfortunately the audio controls on the device are not standard, so I developed a simple daemon and accompanying udev and pm-utils script to get the device to work. I’ve published everything under the GPL hoping that they might be useful for someone else. Feel free to clone and fork my repository on github
Lightspark 0.6.0.1 released
Posted by Alessandro Pignotti in Uncategorized on June 11, 2012
I’m very happy to annouce a new major release for Lightspark, the open source flash player implementation. This release includes quite a lot of fixes, both internal and visible to users. The most important ones from a user point of view are probably the improved support for PulseAudio flat volumes, which makes it impossible for lightspark to manipulate the system volume and the newly added support for the BBC video player. To use BBC site you might need to use AdBlock, which is generally recommended since more often than not flash advertisements are not supported by Lightspark and causes failures in otherwise supported sites.
From the ChangeLog:
* Enable RTMP support by default, requires librtmp
* Fixed support for IEventDispatcher implementation pattern
* Improved serialization robustness
* Improved matrix handling
* Implement string and namespace pooling to reduce memory consumption
* Proper support for private namespaces
* Improved support for fonts
* Support LLVM 3.1
* Fix full volume issue when PulseAudio flat volumes are enabled
* Initial support for AIR desktop applications
* Support for www.bbc.co.uk video player
Source tarball is, as usual available from Launchpad.
iCTF hacking competition 2010: solution of some challenges
Posted by Luca Invernizzi in Uncategorized on December 10, 2010
This year, having joined the computer security group at UCSB for my phd, I’ve helped in the organization of the 2010rh edition of the iCTF, the biggest international online hacking competition. It has been plenty of fun, with more than 70 teams participating from all over the world. The CMU team “Plaid parliament of pwning” won it, getting the 1000$ price (thanks Adobe and IEEE Security & Privacy magazine for the sponsorships!).
Since people have been asking for the solutions of the two challenges I wrote, so here they are.
challenge 5:
This challenge is easy, I encourage you to give it a try, it’s fun!
Question: “Who’re you’re gonna call?”
File: call
Points: 300
Teams that have completed it: 44 (congrats!)
Solution: in an html comment following this line
challenge 4:
This challenge is a little more difficult, but if you know python you have all the skills necessary to beat it.
Question:
To make it run, unpack this file and run server.py (it’s all python, you can check it for backdoors). To start the challenge, you should read only the content of the “pub” directory: that was the material that was given in the ctf.
Points: 500
Teams that have completed it: 0, as most of the difficult challenges — because of the structure of the iCTF 2010, it turned out that it was more convenient to focus only on the easy challenges. We’ll have to fix it next year!
Solution: in an html comment following this line. The given file also contains a script that can solve the challenge (the test_* files)
For the solution of another difficult challenge, head over to Bryce’s blog.
Lightspark 0.4.5 RC is out!
Posted by Alessandro Pignotti in Uncategorized on November 27, 2010
Finally, after a long delay caused by several issues Lightspark 0.4.5 is out. Here it is a brief Changelog.
- Include the new Advanced Graphics Engine, that should provide smoother and faster graphics and support for clipping
- More robust input support (makes it possible to use Play/Pause on YouTube)
The work on this released has been especially slowed down by an issue found in libxml++ and another one found in mesa. Packagers should pay attention to fulfill the following dependency requirements:
- Libxml++ version 2.33.1 or better. If an older version on libxml++ must be supported some commits must be backported. More info about this in the README file
- Mesa should include the fix for this bug. The issue affects some radeon and maybe intel cards.
Packages will be available for Fedora Rawhide and are already uploaded on the PPA for Ubuntu Maverick
See you in a week!
Posted by Alessandro Pignotti in Uncategorized on November 16, 2010
Me and most of my engineering colleagues here at Sant’Anna School for Advanced Studies are leaving tomorrow morning for a week long tour of universities and labs in Belgium and Netherlands. This means I’ll have no time to work on Lightspark, sorry
Anyway the 0.4.5 release will be probably out in the last days of the month. The critical bug in libxml++ is now fixed as my patch was accepted upstream. If (as I believe) a new version of the library will be released and packaged before i get back there will be no reason to hold the release anymore.
Lightspark 0.4.4.2 released
Posted by Alessandro Pignotti in Uncategorized on September 12, 2010
Another week, another bugfix release for Lightspark! Apart from restoring the support for YouTube this release features the new plugin based audio framework that makes it possible to support other backends beside PulseAudio. At the moment both an ALSA and OpenAL plugins are being worked on.
As always you can grab the release here on Launchpad
Getting Things GNOME! — GSoC review (#11)
Posted by Luca Invernizzi in Uncategorized on August 6, 2010
Hello planet!
I’m back from GUADEC. It was my first conference about open source and it was great.
I’ve found particularly inspiring the talk by Guillaume Desmottes about Telepathy and Epiphany, which can be great to extend GTG possibilities in collaboration.
The talk by Jake Edge about promoting free software projects was also very interesting, in particular for the young Lightspark project (that went completely unnoticed for a few months before showing up on planet GNOME).
Thanks to the exciting talks and people at GUADEC, the GTG team (even the people who were not there!) has been working fervently on a nice rewrite of some parts of GTG core, along with a lot of unit-tests. Hopefully, a lot of bugs will be closed thanks to this, and GTG will be nicer to code.
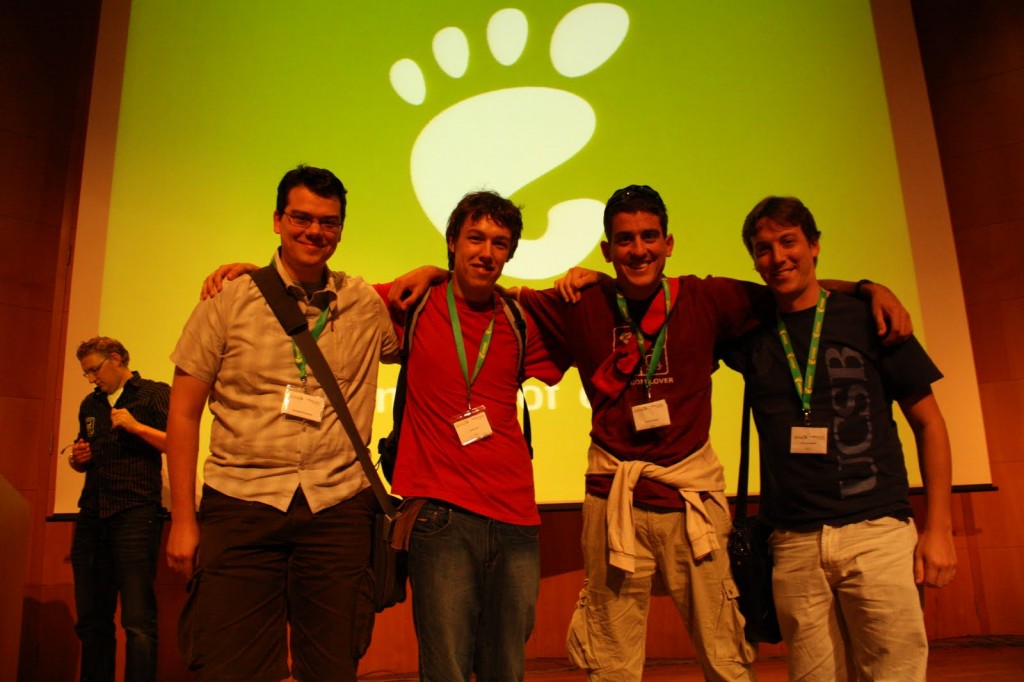
Some of the GTG people at GUADEC. From left to right: Bertrand Rousseau, Karlo Jez, Lionel Dricot and me.
As for my Google Summer of Code on Getting Things Gnome support for multiple backends, this week has seen:
- a port of my Evolution plugin as a backend (that was the last one planned)
- refactoring of the Twitter plugin to get authorization through Oauth (using the tweepy library, thanks Tante for the hint)
- docs, docs, docs
Next week, I’ll keep documenting and testing. I should also write a guide on how to write new backends. See you next week!
Lightspark 0.4.2.2 released
Posted by Alessandro Pignotti in Uncategorized on July 27, 2010
Trying to keep up with the old rule “Release early, release often” I’ happy to announce release 0.4.2.2 open source flash player.
This apparently small point release actually includes the biggest feature plannend for the upcoming 0.4.3 release, namely Gnash fallback on older SWF clips. Lightspark currently relies no Gnash for any Flash content that does not require AVM2 (ActionScript 3) support.
I would also like to explain an issue that many users and testers reported. Firefox is not able to handle multiple plugin for the same file type! Not even if only one of those plugins is actually enabled. So, if lightspark is installed alongside adobe’s player or Gnash no flash content will be displayed. This is a firefox bug, I’ve reported the bug and proposed a patch that is currently waiting the review.
The source of the release is as always available on launchpad. Binary packages for Ubuntu Lucid and Maverick will be available on the usual PPA (in a couple hours from now, Launchpad seems pretty busy at the moment). Moreover, since the last announcement lightspark has been also included in the debian experimental suite (thanks to Didier Raboud and Luca Falavigna).
Stay tuned, and follow the roadmap